Software Processes¶
Based on Chp 1 & 2 of Sommerville “Software Engineering” (9th edition)
Software Processes¶
a software process*, or methodology, is the set of activities you follow to create a piece of software
they are quite important for big, or multi-person software projects
you must decide who does what, and when
you need to be sure you are building the right software!
how do you know the customer will be happy with the completed software?
you also need to know that the software you built is efficient and error free
- or at least as efficient and error-free as necessary to be useful
Example: A Personal Software Process (?)¶
when you work by yourself on a programming assignment, your software process might be simple, implicit (it’s not written it down), and informal
you probably do things like
- decide when to start working on it, and how much time to spend on it (maybe not planned out in detail)
- determine what you are supposed to do i.e. you read the assignment, ask friends questions
- think about the overall structure of the code
- maybe sketch a diagram
- possibly work out an example or two by hand
- write some code, test some code
- repeat the previous steps until it looks done (or you run out of time)
Example: A Personal Software Process (?)¶
- decide when to start working on it, and how much time to spend on it
- we’ll call this part planning and scheduling
- determine what you are supposed to do
- we’ll call this part specification
- think about the overall structure of the code
- we’ll call this part design
- write some code, test some code
- we’ll call this part implementation and validation (or testing)
Example: A Personal Software Process (?)¶
now lets abstract this process
- planning and scheduling
- specification
- design
- implementation and validation (testing)
one very common aspect of software development missing in most computer science assignments: evolution (maintenance)
when you finish an assignment you typically abandon it and don’t update or change it
in real life, software evolution/maintenance is often the longest and most costly part of its life
Basic Software Process Elements¶
- planning and scheduling
- specification/requirements
- design
- implementation and validation (or testing)
- evolution (maintenance)
a software process (or software methodology):
- provides more details for each of these elements
- explains how they connect to each other
- may provide tools and techniques for accomplishing them
Personal Software Process¶
- planning and scheduling
- begin/end dates provided by the assignment
- but you decide when exactly you will work on the assignment, and for how long
- specification/requirements
- often the assignment provides this for you
- puzzle-style programming questions may have extremely precise specifications
- more open-ended project-style assignments may have intentionally vague specification that require you to fill in the details
- some projects may have extremely vague specifications, e.g. little more than “make a game”
- design
- might be able to do this in your head and by hacking code, e.g. getting a general idea of how to proceed and trying it out
- perhaps get some ideas by doing some research, e.g. searching the web, talking to other students, reading the textbook
- sometimes might be useful to draw diagrams, or work through examples
- implementation and validation (or testing)
- start type in the language you are required to use
- often a very small amount of testing, e.g. checking if one or two common cases work correctly
- evolution (maintenance)
- usually none!
The Waterfall Model¶
perhaps the most basic software process is called the waterfall model
it essentially says
do the basic phases in the order they occur, one after the other, and carefully plan everything out at the start of the project to avoid problems
because of the emphasis on planning, there is often a lot of documentation associated with waterfall model
practically, there must also be some way to re-visit past phases when mistakes occur, changes are made, or new things are learned
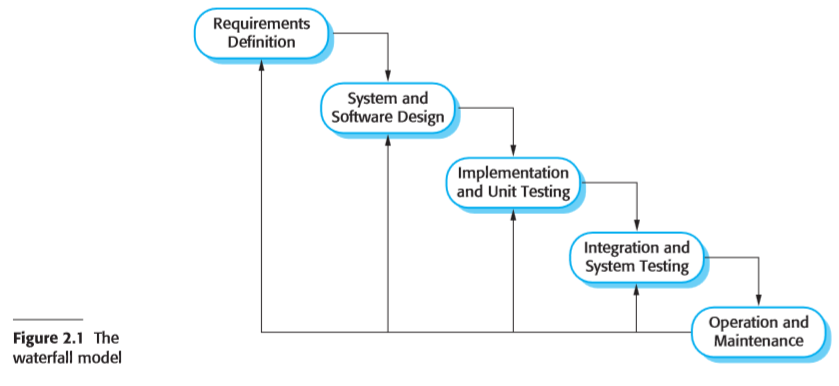
Waterfall Model¶
- good things about the waterfall model
- it fits well with other (non software) engineering processes
- its simple and straightforward, even for non-technical people
- easy to put begin/end dates on the phases (at least as compared to other software processes)
- emphasis on planning and documentation encourages you to think things through
- the best way to deal with a problem is to avoid it completely!
- can be good for very large projects, where a clear and simple process is extremely important
- not so good things about the waterfall model
- if the planning is not well done, could be a disaster
- planning is very hard to do well
- needs lots of care and lots of experience
- plans are usually created at the start of a project when you have the least information available to make a good plan!
- large amounts of documentation can be a burden
- documentation can be tedious to write
- time and effort are needed to keep it updated
- otherwise it might end up being ignored: why create documents no one cares about?
- doing phases in order one after the other is inflexible and too simple
- in practice different parts of a project will be in different phases at the same time
- leaves testing to the end, which is often too late
- if the planning is not well done, could be a disaster
Incremental Development¶
in practice, it is usually better to do some sort of incremental development
basically, divide your project into many smaller “releases”, or “versions”
each small release goes through its own little mini-waterfall process (or something like it)
the software “grows” incrementally
much easier to plan smaller increments than the entire project
gives you partially-working versions of the system early on that you can learn from and demo to people
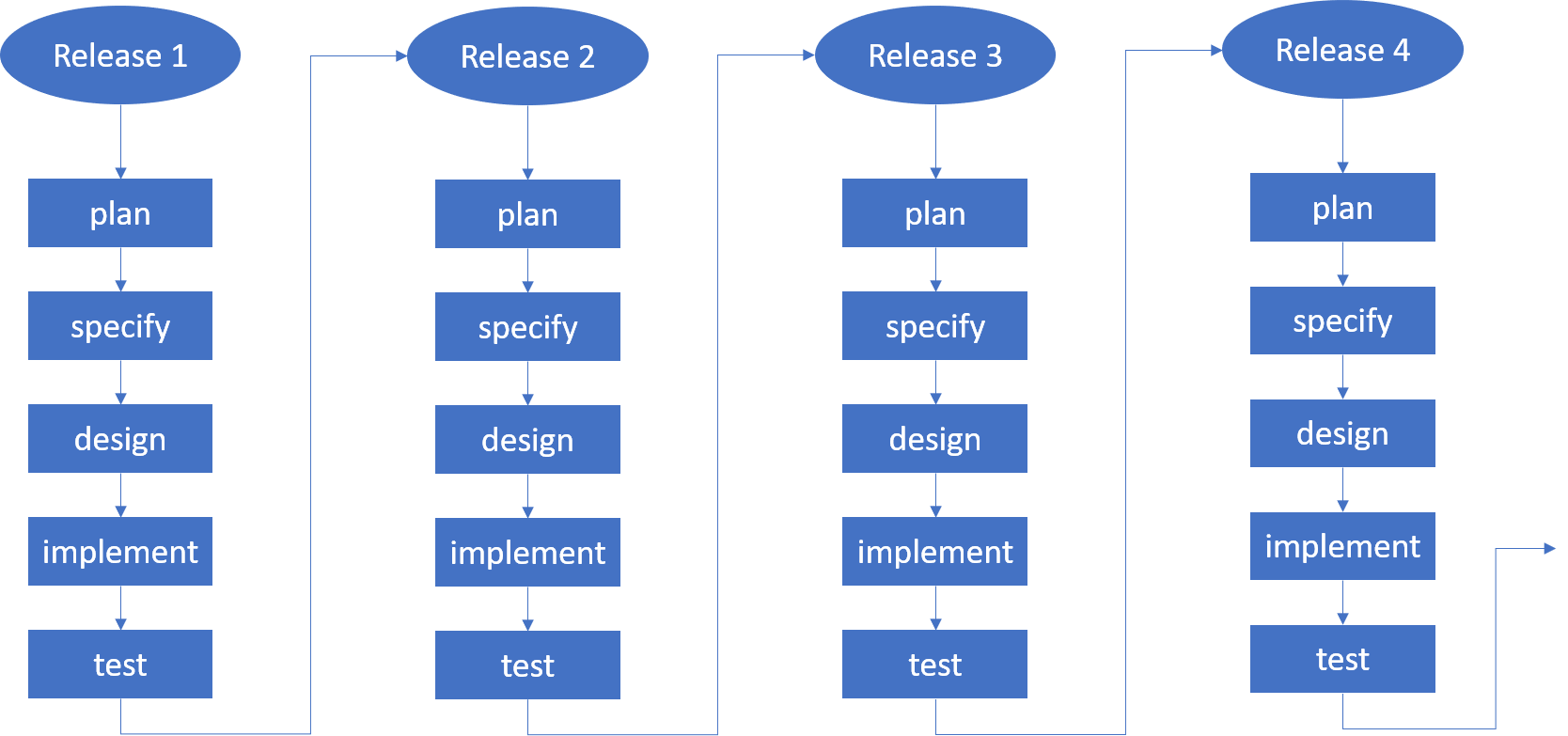
A Note on Reuse-oriented Software Engineering¶
in real life, a business must often make a buy versus build decision for software
for instance:
- should your company buy a word processor (like MS Word), or build their own?
- your company is using a new email server, and wants to transfer all email from the current server onto the new one; should they buy software that does this, or build their own?
the Sommerville textbook mentions reuse-oriented software engineering on par with the waterfall model and incremental model
reuse-oriented software engineering uses pre-made “off the shelf” software components, as opposed to writing code from scratch
implementation is much less work
- although configuration could be complex!
- i.e. learning and setting all the options for pre-made software
still need planning, requirements, testing
- building your own software
- is fun!
- can provide the exact features you want
- is expensive and time-consuming
- can have uncertain outcomes
- i.e. what you plan to build might might not always be what you end up actually building
- buying software
- already built
- configuration and installation may be tricky
- you get less say in the exact features
- limits on customization
- update and maintenance of the software is up to the company you bought it
from
- company that makes the software could focus on features that aren’t important to you
- it’s not unheard of for a company to abandon software, or even to go out of business
- already built
we’re going to focus on the “build” aspect of software engineering in this course, so we will have little to say about reuse-oriented software engineering as a general methodology.
Phases in More Detail¶
each high-level phase of a software methodology can be broken down into sub-parts
different methodologies do this break-down differently
there is no “best” or “right” way to do things
it depends very much on the project, people involved, “corporate culture”
(corporate culture refers to the way things are generally done by an organization)
Software Specification¶
the idea of the diagram below is to help you decide what to do and what to produce during the specification phase
if your boss tells you to “create a spec”, then reviewing this diagram would be a good start!
specific methodologies are typically more precise about how this should be done
e.g. some methodologies may provide templates for specifications
or require that a prototype be used
etc.
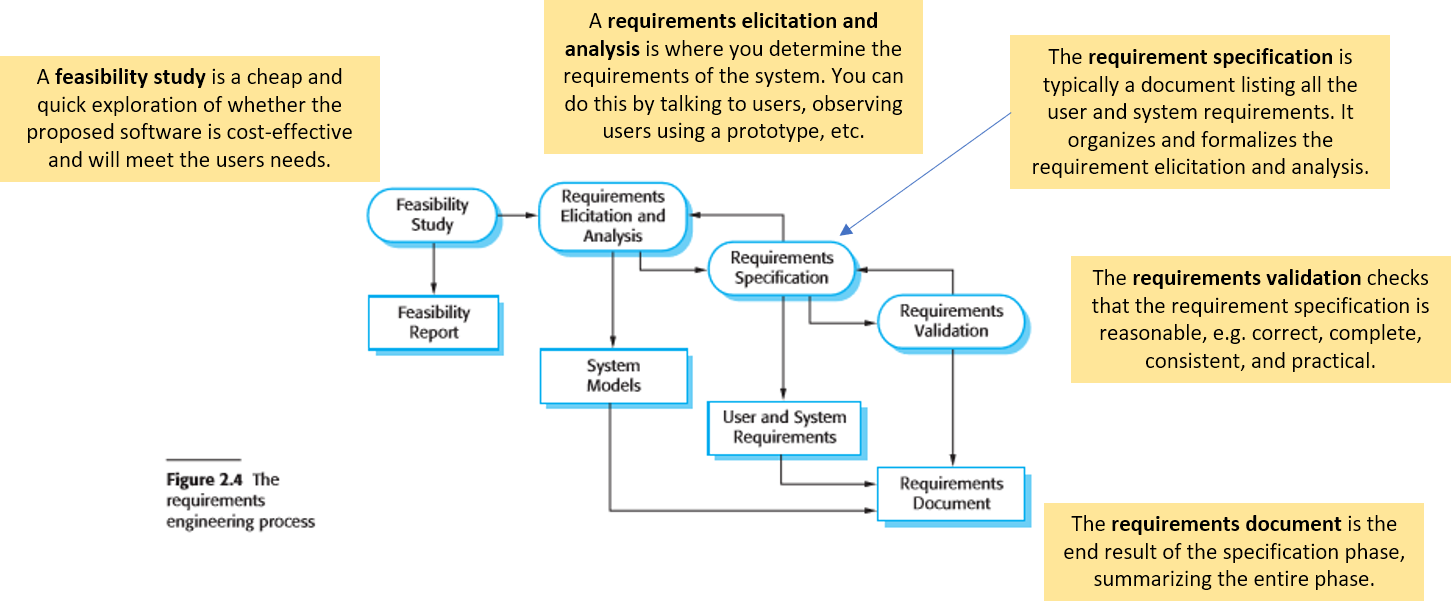
Design¶
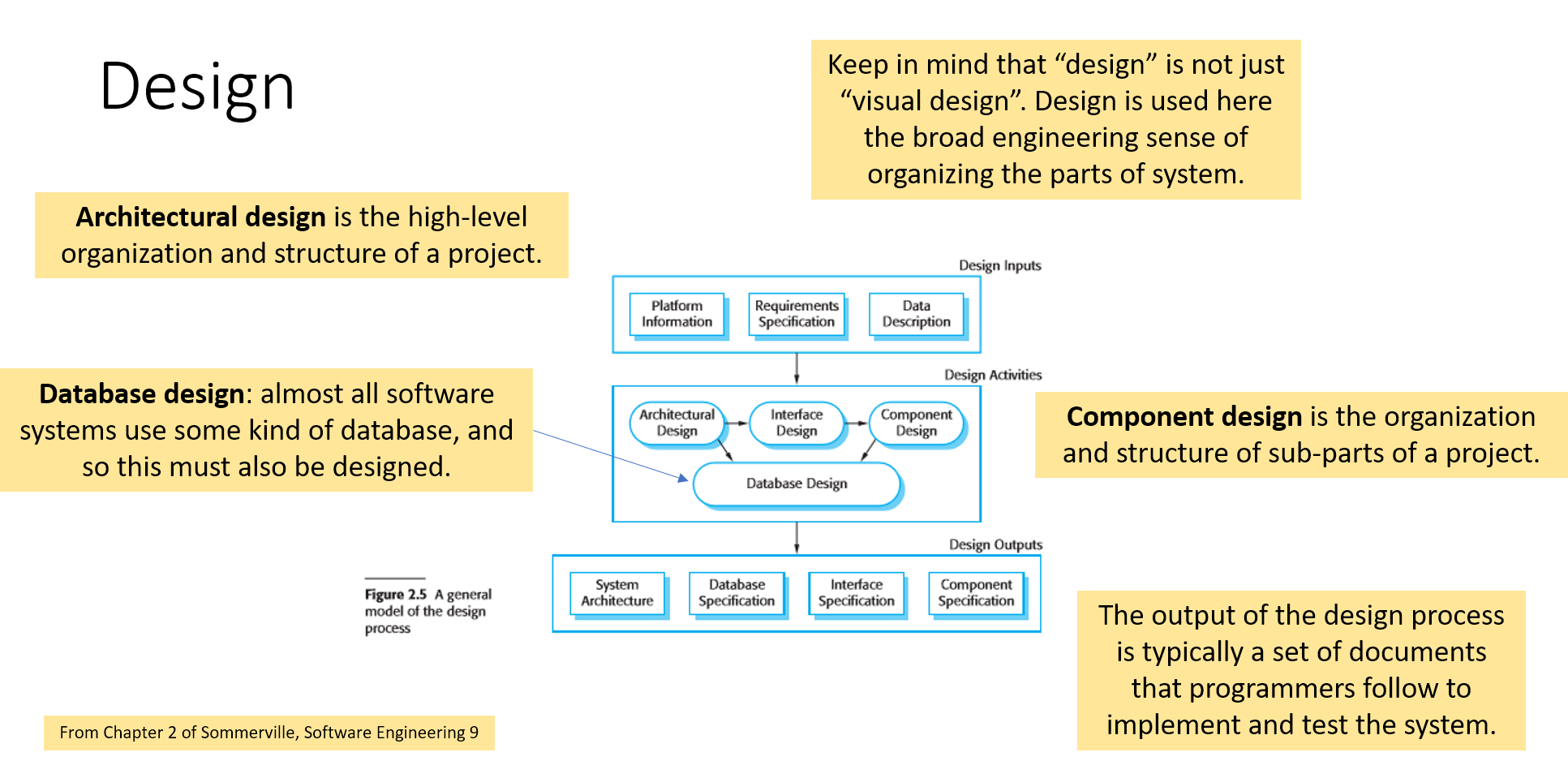
Validation (Testing)¶
validation is about checking to ensure a system meets its specification
does the system do everything that the specification said it would?
does it do these things correctly?
the most common validation technique is testing i.e. running the system (or a sub-part of it) on sample data
there are three main kinds of testing
Unit testing
- testing a sub-part of the system
- often done by the programmer as they create code
System testing
- testing the entire system
Acceptance testing
- testing if users like the system by running it on data from real users
Validation (Testing)¶
suppose you are validating an email client
Unit testing (small)
- individual programmers write tests as they code
- use a testing framework to organize their tests (e.g. JUnit, Google Test, …)
System testing (medium)
- when a usable version of the entire system is ready, the team starts to test the entire system
- they do it by using it: “Eat your own dog food”
- project manager arranges these tests
Acceptance testing (large)
- when a version of the system is usable enough for real users, actual users are invited to try out the system
- developers observe and interview users to see what they liked and didn’t like
Software Evolution (Maintenance)¶
after software is released, it needs to be maintained and upgraded
bugs are fixed (hopefully!)
new features added
surrounding programs might change, requiring changes to the software e.g. operating system update
if the software is successful, this is often the longest phase of its life
traditionally, development and evolution were thought of as distinct activities
Stereotype: as compared to development, maintenance activities are boring and less challenging
that stereotype is no longer accurate in many cases
projects that mix development and evolution are more and more common
e.g. early access games let people buy and play early version of games
e.g. web-based software may let you use early versions of the website, continually adding/fixing features over time
e.g. software subscriptions where you pay a monthly/yearly fee, and get all updates and fixes as soon as they;re ready