Our First Program¶
In these notes you will learn:
- Enter and run a simple program in the Processing editor.
- How to draw ellipses and rectangles.
- How to determine the position of the mouse.
- How to clear the screen.
Getting Processing¶
Processing is a freely available that comes with an easy-to-use programming. It works on Mac, Linux, and Windows systems.
Note
You can expect Processing to work essentially identically on different systems. Past students have used Processing on all these systems with no almost no noticeable differences. This is a pretty impressive technical achievement that is due to Java: Java designed to make the same Java program run identically on any computer.
Download and install the most recent version of Processing for your computer. We’ll be using the editor that comes with it throughout the course. When you first run it, it should look something like this:
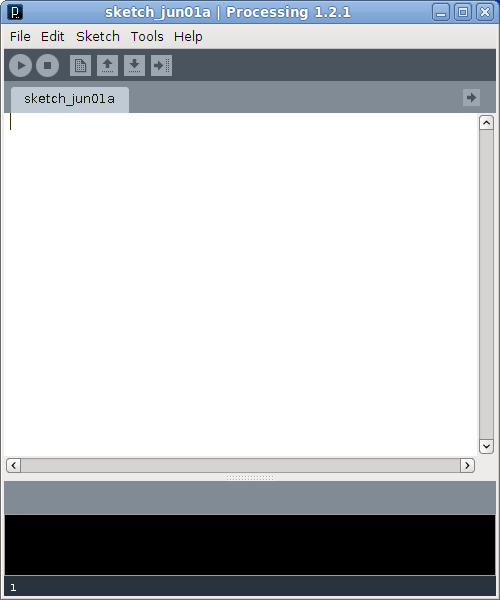
Running Your First Program¶
Lets write our first Processing program. Launch Processing and type the following into the editor window that appears:
void setup() {
size(500, 500);
}
void draw() {
ellipse(mouseX, mouseY, 40, 40);
}
Warning
You must type this program exactly as it appears: a single wrong character could cause it to fail!
Now run the program by clicking the “play” button at the top of the window, i.e. the little triangle in the circle at the top-left of the screen (under the File menu). Move the mouse around, and you should see lots of circles, e.g.:
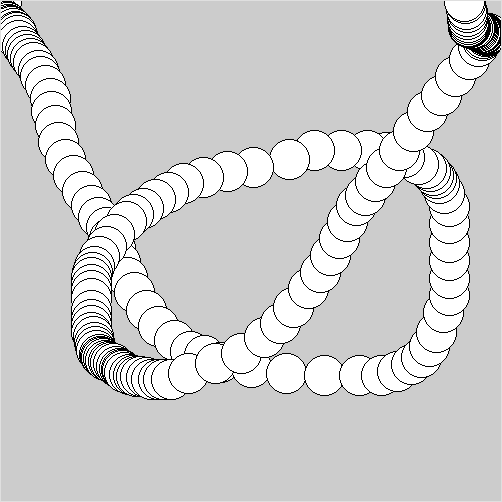
After pressing play we say that the program is running, or executing. This particular program runs until you either click the “stop” button (the little square in the circle), or quit Processing (e.g. by closing the Processing window, or choosing “quit” in the File menu).
Note
To save a program to a file, click the square with the downward-
pointing arrow, or press ctrl-S (i.e. hold down the ctrl key and then press
S). Give your program a descriptive name to help you remember what it is
about, e.g. firstProgram
would be good.
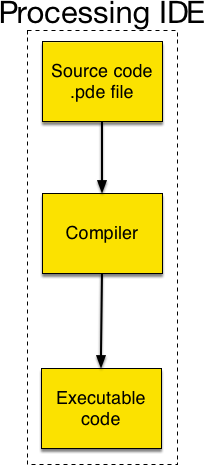
Note
Processing files end with the extension .pde
. This is important
because many programs decide how to handle a file based on its extension.
Examining Our First Program¶
Now lets look in detail at our program. The text of a program is called its source code, or code for short:
void setup() {
size(500, 500);
}
void draw() {
ellipse(mouseX, mouseY, 40, 40);
}
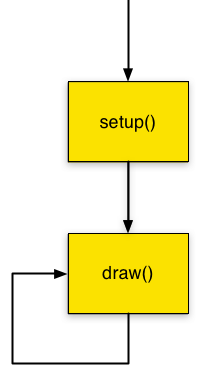
The first thing to note is that the source code is divided into two
functions, one named setup()
and the other named draw()
.
Most Processing programs we write will have these two functions
(and maybe more).
The setup()
function is called precisely once at the start of your
program. When a function is called the code inside of it, i.e. the code
between the open brace {
and the close brace }
, is executed. In this
program, setup()
contains a single function call to size
that sets the
width and height of the output window to be 500 pixels high and 500
pixels wide.
After setup()
finishes, the draw()
function is called repeatedly. That
is, Processing calls draw()
again and again forever. Each time draw()
is called, an ellipse 40 pixels wide and 40 pixels high is
drawn at the position of the mouse pointer, i.e. at location (mouseX
,
mouseY
) on the screen.
A nice way to visualize how setup()
and draw()
are called is shown in
the flow chart on the right.
Both setup()
and draw()
are void
functions, which means they do
not calculate a value the way a regular mathematical function does. Later we
will see that you can write functions that calculate new values, such as
numbers (e.g. a square-root function) or strings.
mouseX
and mouseY
are examples of variables. These
particular variables are pre-defined by Processing and are automatically set
to be the current coordinates of the mouse pointer on the screen.
Frame Rate¶
In one second, Processing calls draw()
approximately 60 times. We call
this the program’s frame rate, and the pre-defined Processing
variable frameRate
is an estimate of a program’s current frame rate. The
number of frames per second that a program runs at is often abbreviated
fps.
If draw()
takes a long time to finish, then the frame rate might be less
than 60. For complex animations that do a lot of work to generate each frame,
it is important to keep an eye on the frame rate to make sure it never dips so
low that it makes the animation look bad.
Indentation¶
The format of source code is quite important because human programmers need to read it. Poorly formatted source code is difficult understand and change.
So, in Processing, like most other languages, we use identation to visually
indicate a block of code. For example, the statement inside
setup()
is indented a couple of spaces:
void setup() {
size(500, 500);
// other lines of code in setup()
// should be indented the same
// amount just like these comments
}
The close brace }
lines up with the v
in void
. If we add any more
statements to setup()
, they should start in the same column as the s
in size
.
The //
symbol indicates a source code comment, i.e. a note for the
person reading the program. We will often add comments to our programs to make
them easier for humans to read.
Note
Processing editor will automatically indent your code for you if you press ctrl-T (hold down the Ctrl key, and then press T).
Playing with the Code: Ellipse in the Middle¶
Now lets play with our program a little bit to see what else it can do. Re- write it as follows (again, be very careful to type the code exactly as given!):
void setup() {
size(500, 500);
}
void draw() {
ellipse(250, 250, mouseX, mouseY);
}
Here we’ve changed the parameters to the ellipse
function: now
the ellipse’s center is always drawm at (250, 250) (the middle of the screen),
and its width is the value of mouseX
and its height is the value of
mouseY
. Move the mouse pointer around while the program is running to see
the effect.
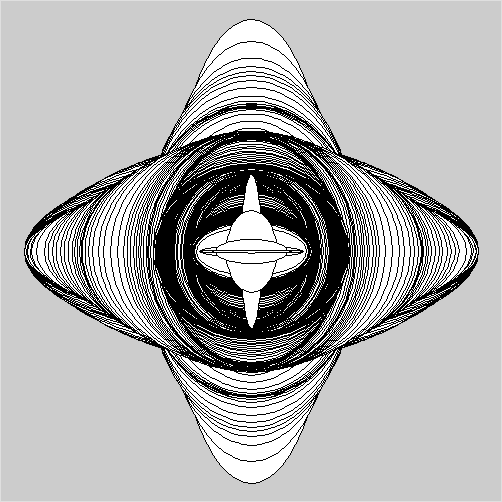
Playing with the Code: Swapping X and Y¶
Here we swap mouseX
and mouseY
:
void setup() {
size(500, 500);
}
void draw() {
ellipse(mouseY, mouseX, 40, 40);
}
This is an unusual effect: it feels as if the mouse is not working properly!
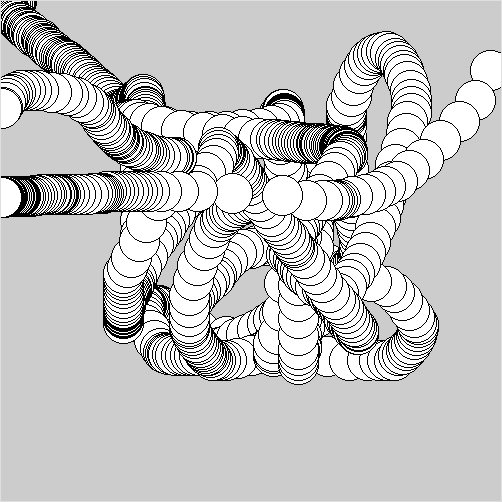
Playing with the Code: Two Ellipses¶
In this program we add another ellipse:
void setup() {
size(500, 500);
}
void draw() {
ellipse(mouseX, mouseY, 40, 40);
ellipse(mouseX + 80, mouseY, 40, 40);
}
The second ellipse is drawn 80 pixels to the right of the first one. If you replace the 80 with, say, 20, then the ellipses will overlap.
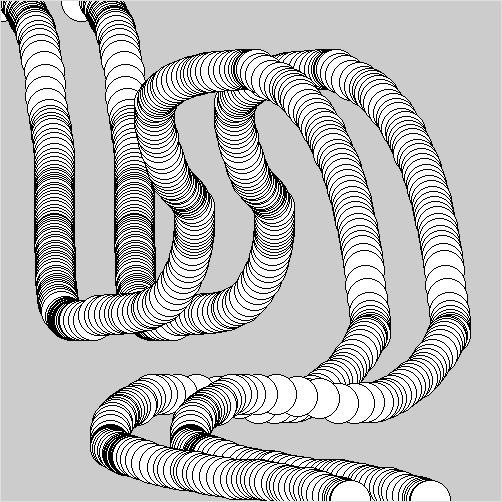
Ellipse Parameters¶
Lets stop for a moment and look in more detail at the ellipse
function.
You call the ellipse
function by writing a statement that looks like
this:
ellipse(x, y, width, height);
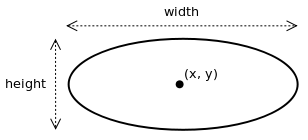
We say that ellipse
has four parameters: x
, y
,
width
, and height
. Each of these parameters must be a number;
width
and height
should both be greater than 0.
Notice a few important details:
- The name of the function is
ellipse
, and after the name comes an open bracket, i.e. a(
character. A matching close-bracket,)
, goes at the end of the line before the;
. - After the
(
bracket we list the function’s parameters. Order matters: first comesx
andy
, which specifies the (x, y) location of the center of the ellipse, and thenwidth
andheight
which specify the vertical and horizontal diameter of the ellipse (not the radius!). - Calling the
ellipse
function is an example of statement, and in Processing a;
character marks the end of a statement.
Almost any other way of calling ellipse
results in an error. For example:
ellipse(0, 0, 0, 250, 250); // too many parameters
ellipse(0, 0, 250); // too few parameters
ellipse(0, 0, 250, 250,); // extra comma
ellipse(0, 0. 250, 250); // . instead of ,
Ellipse(0, 0, 250, 250); // wrong case
elipse(0, 0, 250, 250); // wrong spelling
ellipse(0, 0, 250, 250) // missing ;
ellipse(0, 0, 250, 250; // missing )
ellipse
is a pre-defined function that comes with Processing, and you can
read about it here. The
Processing reference page lists all the
Processing functions, and we will often be referring to it. Bookmark it!
An Important Trick: Re-drawing the Background¶
Now lets make a small but important modification to our original program:
void setup() {
size(500, 500);
}
void draw() {
background(200);
ellipse(mouseX, mouseY, 80, 80);
}
Every time draw()
is called the background(200)
sets the color of the
drawing window to be 200, which us a light shade of gray (a value closer to 0
would make it darker). This erases everything on the screen. Since draw()
is called about 60 times a second, the ellipse appears to move smoothly around
without leaving a trail.
This simple trick of re-drawing the screen background at the start of every
call to draw
is a key idea in computer animation. We’ll use it throughout
this course as the basic technique for getting things to move on the screen.
Questions¶
What is source code?
Draw a flow chart showing when the
setup()
anddraw()
functions are called.Describe, in English, what the following Processing statement does:
size(500, 250);
Write a Processing statement that draws an ellipse of width 50 and height 75 at the current mouse location.
Name two variables that Processing provides.
What is the purpose of a
;
(semi-colon) in a Processing program?What does
//
indicate in a Processing program?
Programming Questions¶
Write a program that draws a square wherever the mouse pointer is on the screen. The square should leave a trail, i.e. do not re-draw the background on each call to
draw()
. Hint: Use the rect function.Re-do the previous question, but this time do not have the square leave a trail, i.e. re-draw the screen background at the start of each call to
draw()
.Write a program that draws a circle inside of a square wherever the mouse points. It should not leave a trail.
Write a program that draws two ellipses both with their centers in the middle of the screen (i.e. at (250, 250) on a 500 x 500 screen). Make the width and height of the two ellipses change in different ways as you move the mouse pointer around the screen. How exactly the ellipses change is up to you.
Read about the triangle function and write a program that makes a triangle follow the mouse pointer around the screen without leaving a trail.
Important: The triangle must keep the same size and orientation as it moves.
Write a program that draws a single line on the screen using the line function. One end of the line should always be at (0, 0), and the other end of the line should be at the mouse pointer. The line should not leave a trail: re-draw the background on each call to
draw()
.Extend the program in the previous question to use four lines, each with one end attached to one of the four corners of the screen. The other end of each line should be at the mouse pointer.