Introduction to Programming with Processing¶
In these notes you will learn:
- What this course is about.
- The pre-requisites for taking this course.
- The basic components of a computer.
- The definition of a programming language.
- Some examples of popular programming languages.
What is this course about?¶
This course is an introduction to computer programming using the Processing language. You don’t need to know anything about programming to take this course. But we do assume you:
- Have interest in learning how to program.
- Enjoy playing with graphics and animation. You don’t need any artistic skill, just a willingness to learn about basic 2D-animation and graphics.
- Know high school algebra. We’ll be using things like (x, y) coordinates, and few other mathematical ideas that should be no problem for you if you’ve taken a high-school algebra course.
Computer Programming¶
Computers are everywhere, and they all run programs. A program is a sequence of instructions that tell the computer what to do, and a programmers job is to create and arrange these instructions. Software refers to a collection of programs.
Programs are usually written to solve a particular problem. For example, a word processor solves the problem of editing documents, and a web browser solves the problem of conveniently accessing files on other computers.
We’ll start with very basic programs, and work our way up to object-oriented programming (OOP).
What is a Computer?¶
Since programs run on computers, it’s useful spend some time exploring what a computer actually is. All computers have, at least, the following basic components:
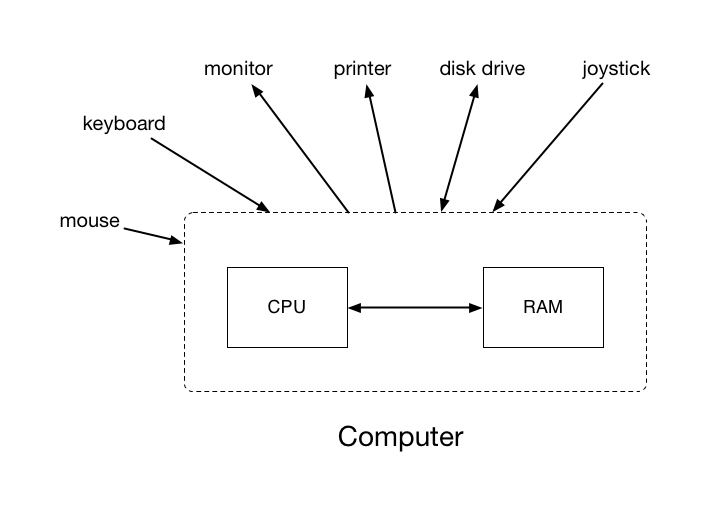
A central processing unit, known as the CPU. The CPU is the so- called “brain” of the computer that makes logical decisions, does arithmetic, and manipulates the computer’s memory.
Note: These days, many PCs have more than one CPU (or core). Programming multiple-CPU computers is much more challenging than programming single- CPU computers because of the difficulty of coordinating the CPUs. We will stick to single-CPU programming in this course.
Random-access memory, known as RAM. RAM is where computers can store and retrieve data. At a very low level, you can think of RAM as a sequence of 0s and 1s (bits), although in this course we will rarely, if ever, need to be concerned with such low-level details. Instead, we will imagine that the computer stores data as numbers and strings of characters.
An important feature of RAM is its speed: data in RAM can be stored and retrieved very efficiently.
Input and output devices, known as I/O devices. Input devices — such as mice, keyboards, touchscreens, disk drives, and video cameras, etc. — let us put data into a computer. Output devices — such as monitors, speakers, printers, and disk drives, etc. — extract data from the computer.
Some devices, such as a disk drive, allow for both input and output.
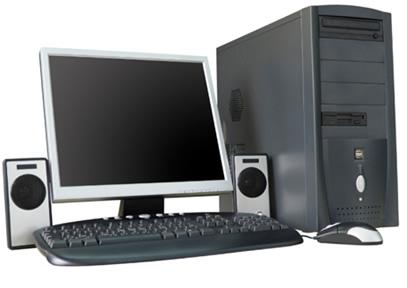
By varying these three elements, you can get different types of computers. For instance, a typical desktop computer might have these features:
- A fast CPU that might do, say, 2.5 billion low-level operations (such as additions or multiplications) per second. Generally speaking, if a CPU runs at 1 gigahertz, then it does about 1 billion low-level operations per second.
- Lots of RAM, e.g. 4 gigabytes of RAM can store about 8000 color images. A gigabyte is one billion bytes, and a byte is 8 bits (where a bit is a 0 or a 1).
- Input devices: keyboard, mouse, large and fast hard disk.
- Output devices: (large) graphical monitor, large and fast hard disk, printer.
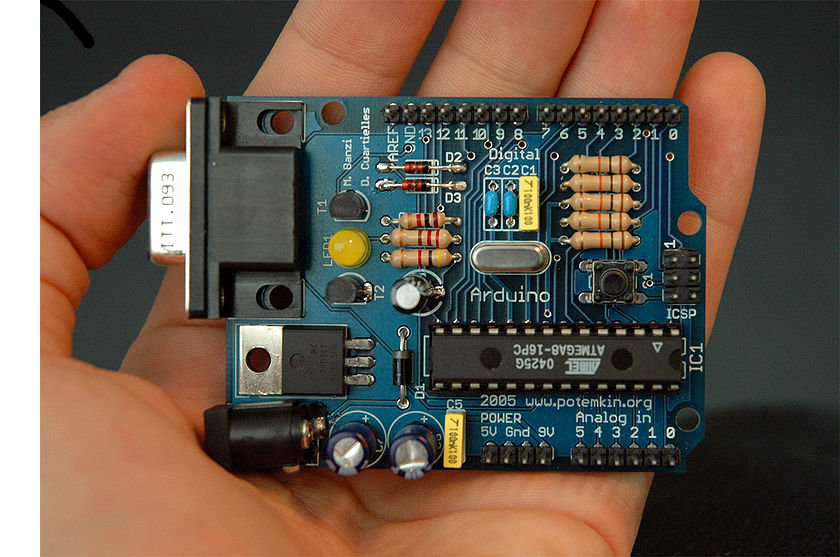
Compare this to a very different kind of computer, the Arduino micro- controller:
One slow CPU that can do about 16 million low-level operations per second. While 16 million is certainly a big number, this is about 150 times slower than a desktop CPU running at 2.6 gigahertz.
In other words, you would have to let the Arduino run for more than 27 minutes for it to do the same amount of work a 2.6 gigahertz CPU could do in 1 second.
About 32 kilobytes of memory. This is enough to store, maybe, one small color image. Maybe.
No input/output devices: instead, the Arduino is designed to control other devices. For instance, you could use an Arduino to control an array of blinking lights.
Note
A fun question to ask is “What is the worlds’s fastest computer?”. While there is no universally agreed upon way to measure the speed of a computer, the website www.top500.org is an interesting list where computers are ranked according to their performance running scientific programs.
What is a Programming Language?¶
To program a computer, you need to give it a sequence of instructions to follow, and these instructions must be written in some sort of language. Since the 1940s when electronic digital computers first appeared, thousands of different programming languages have been created.
The simplest — and usually most difficult — way of programming a computer is to use its assembly language (or just assembly, or assembler, for short). Assembly language programs directly control the CPU and RAM. For example, here’s an assembly language program prints “Hello, World” on the screen:
SEGMT SEGMENT
ASSUME CS:SEGMT, DS:SEGMT
ORG 100h
Main:
MOV AH,09h
MOV DX,OFFSET Text
INT 21h
MOV AX,4C00h
INT 21h
Text:
DB "Hello, World$"
SEGMT ENDS
END Main
Few programmers write programs directly in assembly language. The main problem is that such programs very quickly become long and complex, even for simple tasks. Furthermore, different CPUs have different assembly languages.
So instead, most programmers use high-level programming languages that are easier to read and write.
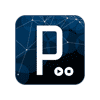
In this course, we will use Processing, a high-level programming language (it’s logo is on the right). Processing is essentially the popular Java programming language combined with a library of useful graphical and animation functions and a handy editor. So by learning Processing, you are really learning Java (remember this when you write your resume!).
Java, and thus Processing, is a high-level language designed for applications programming. Processing is particularly good for creating small interactive graphical programs.
Some Other Programming Languages¶
It is useful to know the names and uses of some other programming languages besides Processing. Here is a brief summary of a few well-known programming languages:
Language Created Purpose FORTRAN 1957 numerics, e.g. scientific computing LISP 1958 symbolics, e.g. artificial intelligence C 1972 systems SmallTalk 1972 education C++ 1983 systems/applications Python 1991 scripting/applications Java 1995 applications JavaScript 1996 web browser language C# 2001 applications Scratch 2006 beginners language Go 2009 systems/applications
While these are all high-level programming languages, they were designed for solving different kinds of problems. For example, C is good for systems programming, e.g. low-level programs that do things like copy files, control disk drives, manage RAM, and so on.
In contrast, application-oriented languages, such as Java, are better for writing programs that will be directly used by people. For instance, Java would be a good language for writing an email reader, or a smartphone game.
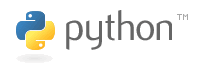
Another popular style of programming language is a scripting language.
Python, for instance, is designed in large part
for writing programs that automate common tasks. For instance, suppose you
have a folder containing 1000 image files ending in .jpeg
that you want to
change to .jpg
. Python would be a good choice for writing a program to
automatically rename all these files since it comes with many helpful built-in
that make accessing files and manipulating strings quite easy.
Questions¶
- What is a program? What is software?
- Draw, and label, a diagram that shows the three main components of a computer system and how they relate.
- What do the following stand for?
- CPU
- RAM
- I/O
- About how many low-level operations can a 2 gigahertz CPU do per second?
- Give examples of I/O devices that are:
- input only
- output only
- both input and output
- What is assembly language?
- What is one good thing about assembly language? What is one bad thing about it?
- True or false: Processing is an example of a high-level programming language.
- How is Processing related to Java?
- What is systems programming? What is applications programming? What is scripting?
- Name two different programming languages often used for writing low-level programs such as operating systems.
- Name a programming language often used for scripting.