Strings¶
In these notes you will learn:
- What string literals are.
- How to use special escape characters in a string.
- How to test if two strings are the same.
- How to combine strings with concatenation.
- How to represent characters with the
char
type.
Introduction¶
In Processing, a string is a sequence of 0 or more characters. For example, these are all strings:
"mouse"
"Once upon a time ..."
"604-555-3536"
"X"
"1\ntwo\nthree"
"Her name is \"Mary\""
"\\n is a return character"
""
More precisely, these are string literals because they begin and end
with a "
(double-quote).
The string ""
is known as the empty string: it is the string
with 0 characters in it.
Strings can contain escape characters in them, such as \n
,
\t
, \\
, and \"
. For example, \n
represents a newline
character. When a newline is printed it makes the cursor go to the next line.
For example, println("hello\nthere")
prints this:
hello
there
We can also use assign strings to variables. For example:
String greeting = "Hello there!";
println(greeting);
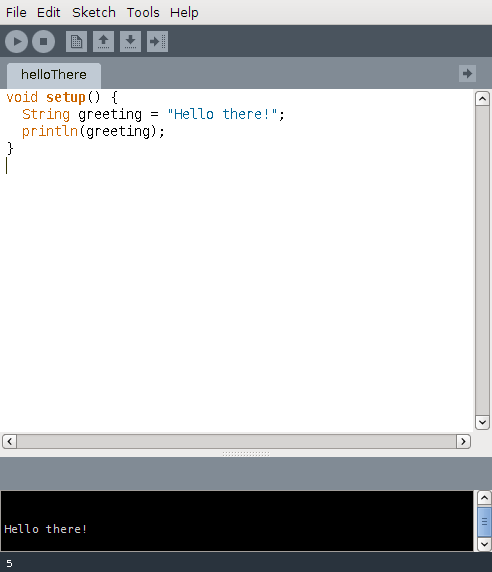
The println
function prints a string on the console; in the Processing
IDE this is the small black window at the bottom of the editor window.
String
is an example of a type. Declaring a variable to be of type
String
ensures that Processing only allows it to store strings. For
example:
String s = "apple";
s = 55; // error: can't assign a number to a String
Notice also that String
begins with a capital S
. If you wrote
string
instead you’d get an error.
Processing provides numerous special functions for dealing with strings. For now we will look at only a few of the most useful ones.
The Length of a String¶
To get the number of characters in a string call the .length()
function.
For example:
String fruit = "lemon or apple";
println(fruit.length()); // prints 14
14 is printed because there are exactly 14 characters in the string lemon or
apple
. Note that spaces count as characters.
You can also call length()
directly on a string literal, although it is
rare in practice:
println("Main Window".length()); // prints 11
Be careful with strings that contain escape characters. An escape character counts as one character, even though it is written with more then one symbol. For example:
String s = "a\nb";
println(s.length()); // prints 3
The string "a\nb"
contains exactly three characters (not 4!): a
,
\n
, and b
.
The empty string has length 0:
String s = "";
println(s.length()); // prints 0
Be careful not to confuse the empty string with a string that contains a space:
String s = " "; // s contains a space character
println(s.length()); // prints 1
Testing if Two Strings are the Same¶
Sometimes you need to know if two string variables, say s
and t
, are
equal. By equal we mean that they have the same characters in the same order.
The .equals
function gives the answer:
if (s.equals(t)) {
println("s and t are the same");
} else {
println("s and t are different");
}
String comparisons are case-sensitive, i.e. .equals
considers "apple"
and "Apple"
to be different.
Warning
Unfortunately, Processing also lets you write code like this:
if (s == t) {
println("s and t are the same");
} else {
println("s and t are different");
}
This does not test if s
and t
are the same strings. While
==
is how you compare primitive values (like int
s and
float
s), it does not work correctly for objects like String
.
For strings, s == t
returns true
just when the variable s
and
the variable t
refer to the same internal memory address. While this
might sometimes give the same results as using .equals
, there are no
guarantees. Generally, comparing strings with ==
is rarely useful, and
so it is almost always a mistake.
Concatenation: Combining Strings¶
Processing lets you create new strings by adding two or more strings together. This is known as string concatenation. For example:
String firstName = "Donald ";
String lastName = "Knuth";
String fullName = firstName + lastName; // "Donald Knuth"
Adding strings together is one way to create formatted output:
String name = "Jay";
println("Hello " + name + "! How are you today?");
// prints: "Hello Jay! How are you today?
Processing also lets you concatenate primitive values (such as float
s)
to strings. For instance:
float x = 250;
println("x = " + x); // prints: "x = 250"
The expression "x = " + x
is interesting because it is a float
added
to a String
. Processing automatically converts the float
x
to a
String
, and then performs concatenation.
You can use the +=
operator to append a string to the end of a string. For
example:
String muppet = "Bert";
muppet += " and Ernie"; // "Bert and Ernie"
Characters¶
Processing also has a data type called char
that represents single
characters. For example, these are all character literals:
'A' 'a' '7' '=' '\'' '\n'
There is no such thing as an empty character, i.e. ''
is an error.
While characters might look like strings, they are not: 'a'
and "A"
are completely different types, and so you can’t, for instance, directly
compare them.
Here’s a simple example of comparing two characters:
char a = 'q';
char b = 'Q';
if (a == b) {
println("same");
} else {
println("different");
}
You must use ==
to compare characters, not .equals
(that would give an
error).
You can access individual charactes of a String
using the charAt
function, e.g.:
String s = "apple";
char firstChar = s.charAt(0); // 'a'
char secondChar = s.charAt(1); // 'p'
char thirdChar = s.charAt(2); // 'p'
The number given to charAt
is the index of the character you would like to
retrieve. The first character of a string is always at index location 0, the
second is at index location 1, and so on.
There’s a lot more that we could say about characters (and strings). However, we will not be using characters much, if at all, in this course. Strings of length 1 work well enough most of the time.
Questions¶
- What is a string?
- What is the empty string?
- Where does
println(s)
prints
? - What is the newline character?
- What does
println("1\n2\n3")
print? - How do you determine the length of a string
s
? - How do you test if the strings
s
andt
are the same (i.e. they have the same characters in the same order)? - Why is comparing strings with
==
a problem? - What is string concatenation?
- What is the value of the expression
"1" + "2"
? - What is the value of the expression
"1" + 2
? - Give an example of how the
+=
operator works with strings. - Explain the difference between
'a'
and"a"
. - How would you test if the characters (both of type
char
)x
andy
are equal?
Programming Questions¶
Write a program that makes a ball bounce around the screen and modify it so that every time you click on the screen with the mouse, this message is immediately printed on the console window:
mouse click at (x, y)
Replace x and y with the coordinates of where the mouse pointer actually was when it was clicked.
Make sure the console message is exactly the same format as in the example!