A Little Bit about For-loopsΒΆ
a loop is a programming language features that repeats a block of code some number of times
for example, this is a JavaScript for-loop that prints Hello 5 times on the console:
for(let i = 0; i < 5; ++i) {
console.log('Hello')
}
loops can be represented visually as flow charts, e.g.
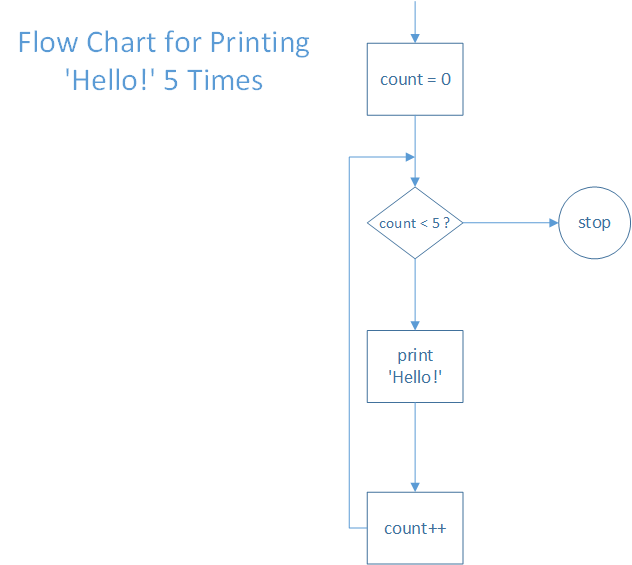
here’s another for-loop that prints the numbers from 1 to 10 on the screen:
for(let i = 1; i <= 10; ++i) {
console.log(i)
}
recall that ++i
increments i
by 1; you can replace that with some
other increment, e.g.:
for(let i = 1; i <= 10; i += 2) { // i += 2 increments i by 2
console.log(i)
}
the above for-loop prints this:
1
3
4
7
9
this for-loop prints the numbers from 10 down to 1:
for(let i = 10; i >= 1; --i) {
console.log(i)
}
in general, this kind of for-loop has this format:
for(let i = init_val; stopping_test; increment) {
// ... loop body ...
}
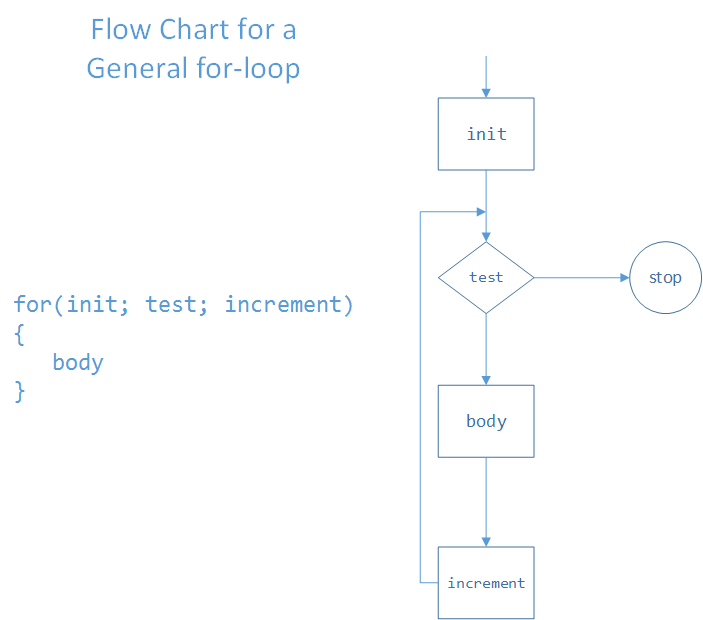
the variable i
is the traditional variable used in a for-loop, and is
short for “index”; you could use any other (sensible!) variable name instead
of i
if you prefer
the stopping_test
is any boolean expression that evaluates to either
true
or false
; it usually somehow involves i
(as in the examples
above)
the increment
is how i
will be updated at the end of each execution of
the loop body; this is usually a statement like ++i
, i+=2
, --i
,
etc.
if you want to repeat a block of code, say, 100 times, then this for-loop is a standard way to do that:
for(let i = 0; i < 100; ++i) { // repeats the loop body 100 times
// ... loop body ...
}
it’s important to notice that i
is initialized to 0 (not 1), and the
stopping test is i < 100
(not i <= 100
)
starting at 0 is quite common in programming, especially in for-loops, and it works well with other parts of JavaScript