Project 3¶
For this assignment, your task is to create an animated analog clock, similar in style to a traditional wall clock.
The questions that follow refer to this picture of a typical wall clock. When your program is done, it should look somewhat like this:
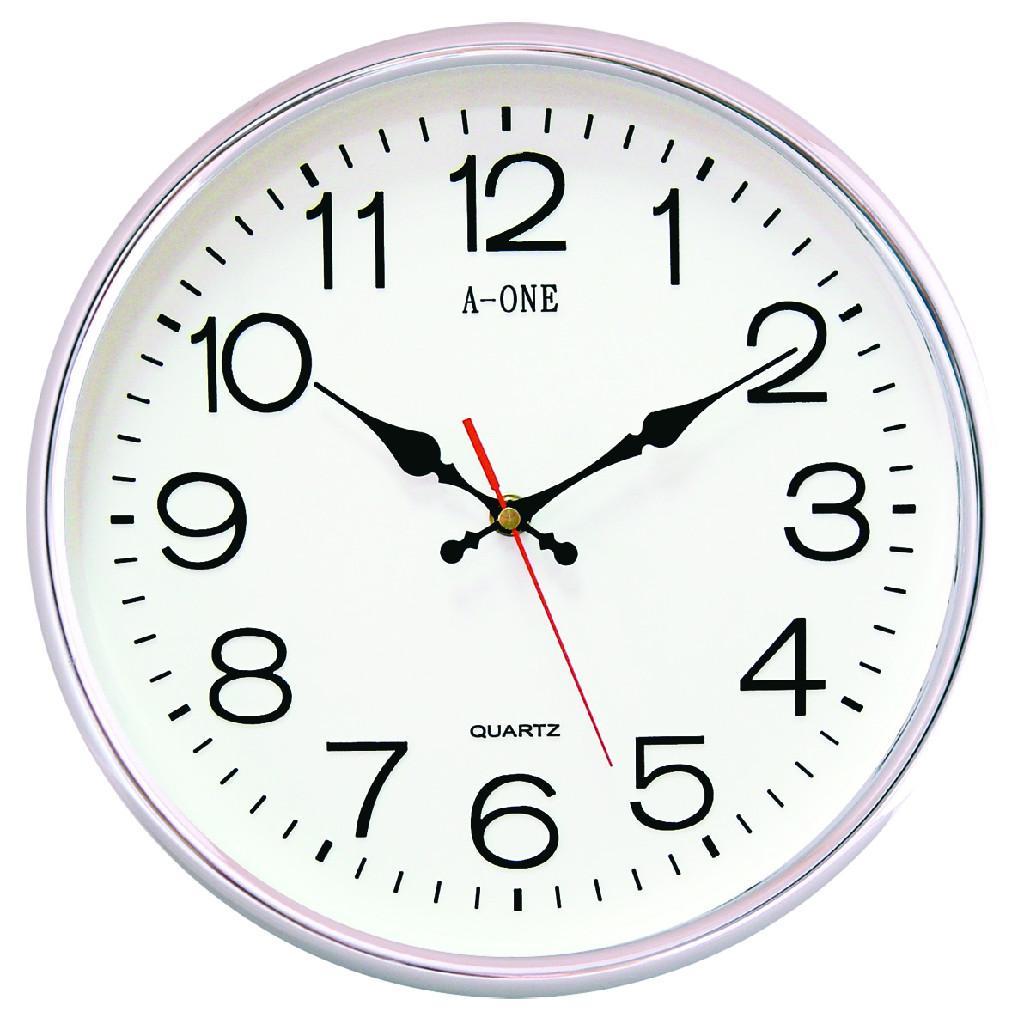
Of course, the hands of your clock should move and display the correct time!
To help you get started, we’ve provided some the code at the end of this assignment that you should copy and modify.
(1 mark) Make the starting code for the clock more modular by putting the drawing code from
draw()
into separate functions.First, replace the
draw()
function with this:void draw() { background(255); // get the current time int s = second(); // values from 0 - 59 int m = minute(); // values from 0 - 59 int h = hour(); // values from 0 - 23 // move origin to center of the screen translate(250, 250); drawSecondsHand(s); drawMinutesHand(s, m); drawHoursHand(s, m, h); drawCenterPin(); }
For this to work, you will need to create the following four
void
functions:drawSecondsHand(s)
drawMinutesHand(s, m)
drawHoursHand(s, m, h)
drawCenterPin()
When this is done, the program should behave exactly the same, but now the source code is easier to read.
(1 mark) Add a feature to the program that “toggles” the seconds-hand. If the user presses the S key when the seconds-hand is displayed, the seconds-hand disappears (and the other hands remain visible). When the seconds-hand is not displayed, pressing the S key makes it re-appear.
Make sure your program handles both lowercase s and uppercase S.
(1 mark) Create a new function called
drawName()
that puts your name in the same location as the words “A-ONE” in the clock in the picture (i.e. below the 12), and puts “CMPT 166” at the same position as “QUARTZ” (i.e. above the 6). The font choice is up to you.The hands of the clock should be drawn on top of this text.
Be sure to call
drawName()
inside thedraw()
function!(1 mark) Create a new function called
drawNumbers()
that draws the numbers from 1 to 12 on the clock face. Position the numbers as carefully as possible so that it looks like a real clock. The font choice is up to you.Be sure to call
drawNumbers()
inside thedraw()
function!(1 mark) Create a new function called
drawTicks()
that draws 60 equally-spaced “tick marks” at every minute around the edge of the clock face. The ticks should appear “above” the numbers as in the picture.Just as in the picture, the tick marks should be short line segments that are drawn at the same angle the seconds-hand would be at if it were on that exact second. This is important!
It’s not necessary to make the tick marks on the hours thicker than the other tick marks (as in the picture).
Be sure to call
drawTicks()
inside thedraw()
function!(1 mark) Make the entire clock bounce around the screen like a ball. When the clock hits an edge, it should bounce off of it at the same angle and continue moving at the same speed. All the components of the clock should move — hands, numbers, text, etc. — and the hands of the clock should continue to move as usual as the clock bounces around. Don’t let any part of the clock go off the screen.
Hints¶
- The questions are given in approximate order of difficulty.
- You don’t need to write any of your own classes for this assignment.
Starting Code¶
void setup() {
size(500, 500);
smooth();
}
void draw() {
background(255);
// get the current time
int s = second(); // values from 0 - 59
int m = minute(); // values from 0 - 59
int h = hour(); // values from 0 - 23
// move origin to center of the screen
translate(250, 250);
// draw the seconds hand
pushMatrix();
rotate(radians(map(s, 0, 59, 0, 360)));
stroke(255, 0, 0);
strokeWeight(1);
line(0, 0, 0, -225);
popMatrix();
// draw the minutes hand
pushMatrix();
rotate(radians(map(m + s / 60.0, 0.0, 60.0, 0.0, 360.0)));
stroke(0, 0, 255);
strokeWeight(5);
line(0, 0, 0, -200);
popMatrix();
// draw the hours hand
pushMatrix();
if (h > 12) h -= 12;
rotate(radians(map(h + m / 60.0, 0.0, 12, 0.0, 360.0)));
strokeWeight(5);
stroke(0, 255, 0);
line(0, 0, 0, -100);
popMatrix();
// "pin" at the center
fill(0);
noStroke();
ellipse(0, 0, 15, 15);
}