Logical Expressions¶
In these notes you will learn:
- How to use relational operators.
- How to use boolean operators.
- How to use if-statements.
Boolean Values¶
There are only two boolean
values: true
and false
. We true
and
false
boolean
literals to distinguish them from boolean
variables
such as this:
boolean offScreen = false;
Here, offScreen
is a boolean
variable, while false
is a
boolean
literal. They both have the value false
.
Any expression that evaluates to a boolean
value is called a
boolean expression. For example:
if (y + 25 >= 499) {
// ...
}
The expression y + 25 >= 499
is a boolean
expression, meaning it
evaluates to either true
or false
, depending upon the value of y
.
In the rest of this note, we will see the basic operators for constructing boolean expressions.
Some Relational Operators¶
Since boolean expressions are so important in programming, let’s discuss them in a little more detail.
We’ve just seen >=
, which compares numbers and means “greater than or
equal to”, e.g. x >= y
is true
just when x
is greater than, or
equal to, y
, and false
otherwise.
Similarly, the expression x <= y
evaluates to true
if x
is less
than, or equal to, y
, and false
otherwise.
Here’s a list a few common boolean expressions. We assume both x
and y
are numbers:
x >= y // is x greater than, or equal to, y ?
x <= y // is x less than, or equal to, y ?
x > y // is x greater than y ?
x < y // is x less than y ?
x == y // is x equal to y ?
x != y // is x not equal to y ?
>=
, <=
, <
, >
, ==
, and !=
are called
relational operators, or comparison operators.
Warning
The expressions x = y
and x == y
are not the
same Processing. The expression x == y
tests if x
and y
have
the same value, while x = y
assigns the value of y
to x
. This
is very different than what = usually means in mathematics: the
mathematical = means the same as ==
.
Warning
You cannot chain relational operators together as in mathematics, i.e. this does not work in Processing:
1 < 2 < 3 // bad!
This is an error. To evaluate such an expression you must write this (where
&&
means “and”):
1 < 2 && 2 < 3 // okay!
Most mainstream programming languages have the same restriction, i.e.
expressions like 1 < 2 < 3
either cause an error, or don’t work the way
you would expect.
Logical Operators¶
We can also use logical operators to combine boolean expressions. For
instance, suppose both a
and b
are boolean expressions. Then the
following are also boolean expressions:
!a // not a; negation of a
a && b // a and b; conjunction of a and b
a || b // a or b; disjunction of a and b
These three logical operators have precise meanings:
!a
evaluates totrue
whena
isfalse
, andfalse
whena
istrue
.a && b
evaluates totrue
just when botha
andb
evaluate totrue
. It evaluates tofalse
if eithera
, orb
, or botha
andb
, arefalse
.a || b
evaluates totrue
whena
istrue
, orb
istrue
, or botha
andb
aretrue
. The only time it isfalse
is when botha
andb
arefalse
.
We can summarize these logical operators using truth-tables. For example, here is the truth table for &&
:
a b a && b false false false false true false true false false true true true
And for ||
:
a b a || b false false false false true true true false true true true true
And !
:
a !a false true true false
Memorize these tables! Evaluating boolean expressions is one of the most common and important things a program does.
If-Statements¶
The if-statements we’ve used so far all have this form:
// ... previous statements ...
if (cond) { // first line is the header
body statements // code between { and } is the "body"
}
// ... following statements ...
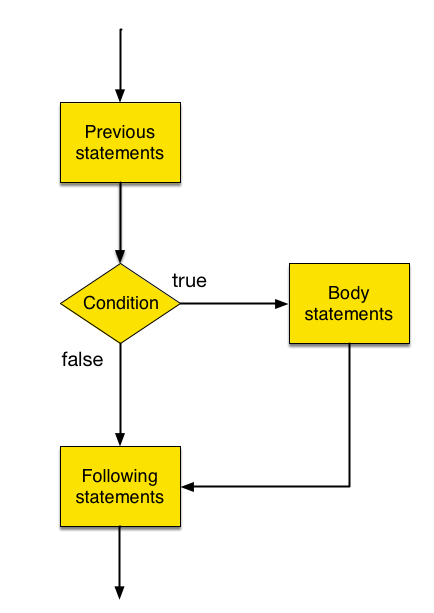
An if-statement begins with the if
token, and is immediately
followed by (cond)
, where cond
is a boolean expression, i.e.
an expression that evaluates to either true
or false
(such as y >=
499
).
The line with the condition is the if-statement’s header line. After that
comes the body of the if-statement. The code in the body is only executed
when cond
is true
; if cond
is false
, then the body is not
executed.
Note
When the body of an if-statement consists of exactly one
statement, it is not necessary to write the {
and }
. For
instance:
if (y >= 499)
y = 0;
Or even more succinctly like this:
if (y >= 499) y = 0;
However, if you are not careful this can lead to confusion about which
statements are part of the body. In this course we may occasionally write
if-statements without the {
and }
. When we do, we will almost
always but the body statement on the same line as the condition.
If-else Statements¶
Another useful form of an if-statement is this:
if (cond) {
A // executed just when cond is true
} else {
B // executed just when cond is false
}
If cond
is true
, then A is executed, and if cond
is false
,
then B is executed.
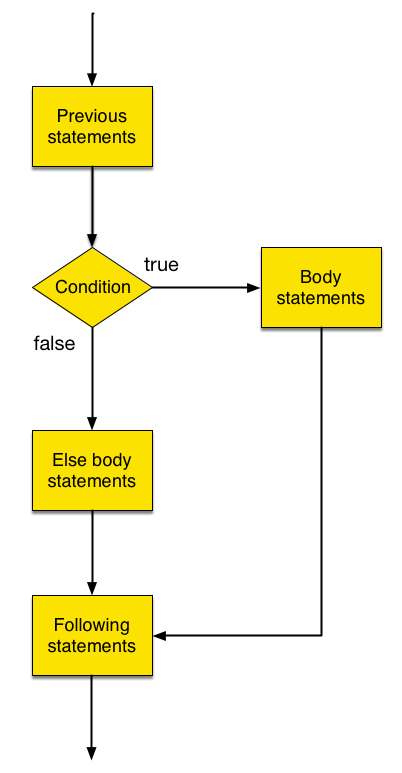
You can even write combine else and if to make statements like this:
if (cond1) {
A // executed just when cond1 is true
} else if (cond2) {
B // executed just when cond1 is false and cond2 is true
} else if (cond3) {
C // executed just when cond1, cond2 are false, and cond3 is true
} else {
D // executed just when cond1, cond2, and cond3 are all false
}
This is the most general form of an if-statement, and sometimes we call it an if-else-if statement.
Some Examples¶
Here’s a simple example of how you might use ||
, i.e. “or”. Instead of
writing y >= 499
in an if-statement, we could write it like this:
if (y == 499 || y > 499) {
y = 499;
}
The expression y == 499 || y > 499
is logically equivalent to y >=
499
. You could write either one in the program, but y >= 499
is less
typing and quite clear, so most programmers prefer it.
As another example, suppose you want to test if y
is between, say, 100 and
200. You could do this:
if (y > 100 && y < 200) {
// ...
}
The expression y > 100 && y < 200
is true just when y
is greater 100
and less then 200.
The !
operator means not. So, for example, to test if y
is not equal
to 499 we could write this:
if (!(y == 499)) {
// ...
}
Equivalently, we could write this using the !=
(not equal) operator:
if (y != 499) {
// ...
}
This second if-statement is usually preferable because it is shorter and
clearer than the one with !
in the front.
A Few Sample Questions¶
Understanding logical expressions is such an important part of programming that it’s useful to work through a few sample questions.
In what follows, assume that x
, y
, and z
are all variables of type
int
. They each have a value, although we don’t know exactly what those
values are.
Write a logical expression that is
true
just when all ofx
,y
, andz
are equal to 5, andfalse
otherwise.One solution:
x == 5 && y == 5 && z == 5
.Note that while you can’t write an expression like this in Processing:
x == y == z == 5
. You must the and operator,&&
, to split this into small parts.Write a logical expression that is
true
just when none ofx
,y
, andz
are equal to 5, andfalse
otherwise.One solution:
x != 5 && y != 5 && z != 5
. In English, you’d read this as “x
is not equal to 5, andy
is not equal to 5, andz
is not equal to 5”.Another solution:
!(x == 5 || y == 5 || z == 5)
. In English, this would be “it’s not the case thatx
equals 5, ory
equals 5, orz
equals 5”.These solutions are logically equivalent, i.e. for the same values of
x
,y
, andz
they will always evaluate to the same thing.Write a logical expression that is
true
just when exactly one ofx
,y
, andz
are equal to 5, andfalse
otherwise.One solution:
(x == 5 && y != 5 && z != 5) || (x != 5 && y == 5 && z == 5) || (x != 5 && y != 5 && z == 5)
This is a rather long and complicated-looking expression, but it’s actually pretty straightforward: it lists all the cases when exactly one of the variables equals 5.
Write a logical expression that is
true
just whenx
,y
, andz
all have the same value, andfalse
otherwise.One solution:
x == y && y == z
.Another solution:
x == y && y == z && x == z
.Notice that the second solution includes the first solution in it. The extra term
x == z
is allowed, but it isn’t necessary becausex
andy
are the same, andy
andz
are the same, then it must also be the case thatx
andz
are the same.Also, the following is incorrect:
x == y == z
. You must use the and operator,&&
to compare pairs of variables.Write a logical expression that is
true
just whenx
,y
, andz
all have different values, andfalse
otherwise.One solution:
x != y && x != z && y != z
.All three terms are necessary here. If you were to leave out, say,
y != z
, then you’d get the expressionx != y && x != z && y != z
, which does not solve the question (e.g. test it withx
equal to 2,y
equal to 2, andz
equal to 3).Another solution:
!(x == y) && !(x == z) && !(y == z)
. What we’ve done here is replace each expression of the forma != b
with the logically equivalent expression!(a == b)
. In English, this says that ifa
andb
are different, then it’s not the case that they are equal.Yet another solution:
!(x == y || x == z || y == z)
. In English, this expression says “it’s not the case any pair of the variables is equal”, which is logically equivalent to say they are all different.Among experienced programmers, the first solution is probably the most common. But the others are okay too, especially if they reflect how you think about what the expression means.
Questions¶
List all possible boolean values.
For each of the following expressions, say whether it is true or false:
1 == 2
1 != 2
1 + 3 < 13
5 > 5
(1 + 2) == (4 - 1)
Suppose
x
andy
are both variables of typeint
. They’ve been assigned different values, but we don’t know exactly what they are. For each of the following expressions, say whether it is true or false:x == y
x != y
x == x
y >= y
(x + y) == (y + x)
Briefly describe the meaning of
!
,&&
, and||
.What are all the possible boolean values of
a
andb
that make the expressiona || b
false
?The expression
(x < y) || (x == y)
can be written as the shorter and logically equivalent expressionx <= y
. Re-write each of the following expression as a shorter equivalent expression:(x > y) || (x == y)
!(x == y)
(x <= y) && (x != y)
!(x == y) && (x >= y)
x != x
For each of the following expressions, state if it is
true
orfalse
. Assume thatx
,y
,dx
, anddy
are defined as follows:float x = 32; float y = -4; float dx = 1.22; float dy = 1.57;
x == x
x == y
dx < dy
(dy > dx) || (dy < dy)
(y == -4) || (x > 0)
(y < -4) || (x <= 0)
(x == y) || (dx == dy) || (x + y == dx + dy)
(1 <= 2) || (x > x)
(x < 0) || (x > 499) || (y < 0) || (y > 499)
(x > mouseX) || (y > mouseY)
Explain, in precise English, what the following code does (the
println
function prints a message on the console window, i.e. on the little window under the source code in the Processing editor):// ... assume x has been defined and given some value ... if (x == 1) { println("Hello!"); } else { println("Goodbye!"); }
Does the following code behave the same as the code in the previous question? Explain your answer.
if (x == 1) { println("Hello!"); } if (x != 1) { println("Goodbye!"); }
Write an if-statement that tests if
x
, afloat
variable that has been given some value (you don’t know what it is) is equal to -2, 7, or 15. Only execute the body of the if-statement ifx
is one of those three values. The if-statements body can just be a source-code comment.Re-do the previous question, except this time only execute the body of the if-statement if
x
is not one of -2, 7, or 15. Give two different answers:- One answer that uses
!=
; - A different answer that uses
!
and either||
or&&
. Don’t uses!=
in this answer.
- One answer that uses