Programs are boring if they just do the same thing every time. We need to respond to things the user does to make things interesting. So far, we have one way to do this: respond to user actions (clicking buttons, or moving the mouse) with jQuery.
That's good but limited: the user clicks on something or they don't. They move the cursor somewhere or they don't. We would like to get richer input from them.
jQuery and Forms
Once we have some input from the user with a form, we can access it with JavaScript and jQuery. The easiest thing to do is find the <input>
with jQuery (probably selecting by id
) and using the jQuery object's .val()
method that will give you what the user typed.
In the example above, we had an input with id="something"
so we can get the string the use typed with $('#something').val()
.
Let's expand that into a more complete example. We can add a button input to the page so the user has something obvious to click to initiate an event:
<p>Type something: <input type="text" id="something" /> <button id="resultbutton">Go</button></p> <p id="result"></p>
When the button is clicked, we will (in show_result
) get what they typed and report it by filling in the content of the <p id="result">
.
show_result = function() { typed = $('#something').val() $('#result').html('You typed this: ' + typed) } setup = function() { $('#resultbutton').click(show_result) } $(document).ready(setup)
Here's what that looks like in the browser, after I typed something and clicked the button:

As before, $('#something')
gives us a jQuery object for the <input … id="something" />
. The .val()
function in that object gives us the string 'Okay I will.'
(in the example above). Once we have the string the user typed, we can use it any way we want.
If you'd like to try it yourself, have a look at the page itself.
Another Example
This time, let's use some form controls and Raphaël: instead of using jQuery to add the text to the page, we'll add it to an SVG.
We will start with a container for the SVG, and the form controls:
<div id="container"></div> <div class="form"> Type something: <input type="text" id="txt-input" /> <button id="add">Add it</button> </div>
We can then use jQuery .val()
to retrieve the text. The Raphaël .text()
function will add it to an SVG. We can also treat it like a string: get its length and use that number to calculate the size of a bar chart-like box.
y = 8 add = function() { entered_text = $('#txt-input').val() len = entered_text.length txt = paper.text(100, y, entered_text) rect = paper.rect(200, y-5, len*8, 10) y = y + 12 } setup = function() { paper = Raphael('container', 400, 100) $('#add').click(add) } $(document).ready(setup)
Here, the variable y
ensures that each time the function add
runs, it draws the text and rectangle further down. The function adds two things: the text itself, and a rectangle with width proportionate to the length of the string. The page will look something like this after a few clicks:
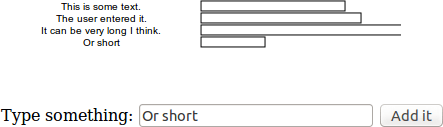
You can try out the example yourself too.
Other Form Controls
The <input />
tag can be used to create other types of controls as well. With all of these, you can use .val()
to retrieve the value, in a way similar to a type="text"
input.
Using type="password"
creates a control that works just like a text input, but where what the user types isn't visible on screen, so they can type a password or other information they wouldn't want visible to someone looking over their shoulder.
Password: <input type="password" id="pw" />
It will look something like this. (These screenshots were taken in Windows 7: the way the widgets look varies between operating systems, but they work the same.)

Other than its on-screen appearance, it works exactly the same as type="text"
: looking at $('#pw').val()
will get the string the user typed.
If you want to do text input where the user has space to enter more text, you can use the <textarea>
tag. You can specify the number of rows and columns (lines and width), and initial text as the contents (or leave it empty for none):
Tell me more: <textarea id="t-area" cols="30" rows="5">This has lots of space for text.</textarea>
It will display like this:
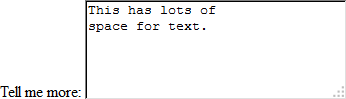
As with the <input type="text">
, you can get the string the user typed with $('#t-area').val()
.
If you have a list of options that you'd like the user to be able to choose, you can use the <select>
and <option>
tags. The <select>
must be used to enclose several <option>
s (like <ul>
contains <li>
s):
Choose one: <select id="seldemo"> <option value="a">Apple</option> <option value="b">Banana</option> <option value="c">Cherry</option> </select>
It will look like this on the page when the user clicks it to make a choice:
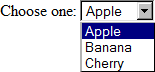
This time when you use $('#seldemo').val()
, you will get the value explicitly given in the <option>
tag. In this example, it will be either the string 'a'
or 'b'
or 'c'
.
There are several other types for <input>
, and other tags that can be used to create form controls. Some of them work a little differently, and some aren't supported as well by browsers. We won't be using others in this course, and you can find all of the details in the HTML Reference.