Step 1: What's my code supposed to do?
If you have any hope of getting your code to work, you'd better know what you expect it to do in order to get the right results.
If you don't know what you want your code to do, take your hands away from the keyboard and figure it out. There's no hope if you don't have a plan for how to get your result.
Ask yourself how the computer can get to the result you want: what steps need to be taken? What needs to happen? What do you need to do with the results?
Once you have that clear for yourself, you can start to figure out why the code you have written isn't doing that.
Step 2: What's my code doing?
Assuming you know what you want your code to do, you can start asking where things diverge. In order to do that, you need to be able to look at see what's happening in your code.
First, if your code doesn't seem to be doing anything, then you may have an error in your code that's preventing the browser from running it. The “Console” tab of your browser's developer tools will show you any errors with your code. (See Browser Developer Tools for more info on the developer tools.). You'll see a description of the error, and the line number in the console.
The easiest way to start diagnosing problems with your logic is the alert()
function, which we have already seen. You can insert an alert()
to confirm that a particular function is running when you expect. Since you can see the alert window and message, you know that line of code ran.
Having a lot of alerts popping up can make it difficult to work with your page, even for debugging. A cleaner alternative is the console.log()
function. This takes whatever you give it as an argument and displays it in the “Console” tab.
For example, if you are trying to connect a click event but can't tell if your code is running, you can add a couple of console.log()
calls like this.
something_click = function() { console.log('something_click running') ⋮ } setup = function() { console.log('setup running') jQuery('#something').click(something_click) } jQuery(document).ready(setup)
You would then hope that after loading the pack and clicking to trigger the event, you would see something like this in the console:
d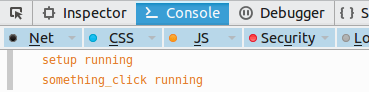
By adding these log outputs, you have learned something: maybe the setup
function never ran and you need to figure out why; maybe something_click
didn't run because the event wasn't connected property; maybe it ran but didn't have the desired outcome. In any case, you have narrowed down where to look for the problem.
If you're unsure on the value of a variable at a certain point, you can log their values as well to see if they are what you expect:
console.log('x and y are...') console.log(x) console.log(y)
You can use these tools (like these examples or other ways) to figure out where the difference is between your expectations and what's actually happening in your code.