There are many CSS properties that you can use: too many to introduce them all right away (or even to remember them all and how they work), but we should see a few more so we can actually do something useful with CSS.
CSS Reference
Like we did with HTML elements and the HTML Reference, we will use a CSS Reference for all of the parts of CSS. You should keep the references in mind, but we can introduce a few key properties now.
We have seen text-align
: have a look at text-align
in the CSS reference. Like the HTML reference, you will see a basic description of the property and the values it can be given.
You will also notice on the reference page for each CSS property a section on “browser support”. This wasn't much of an issue with plan HTML, but with CSS we have to be a little aware of what properties are supported by common web browsers. New features have been added with new CSS versions, and not all are prefectly supported by every browser.
In this course, we will discuss only ones that are well supported, but if you are scanning the reference for others, have a quick look at that the compatibility table to make sure it will work as adveritised.
Useful CSS Properties
Again, we certainly won't be covering every interesting CSS property here, but can introduce a few (with links to the CSS reference for each).
text-align
- As we can seen,
text-align
can be used to change the text justification. Possible values areleft
,right
,center
, andjustify
(for full justification with to ragged left or right margin). font-style
- Used to control italic text: possible values include
italic
andnormal
(for non-italics). font-weight
- Used to control bold text: possible values include
bold
andnormal
(for non-bold). color
- Used to set the colour (usually of the text) for the element. For example, “
color: green;
”. We will discuss colour values more in Colours in CSS. background-color
- Used to set the background colour (behind the text) for the element. For example, “
background-color: black;
”. border-width
border-style
border-color
- These are used to control the border around an element (width of the line, type of line, and colour of line, respectively). You can either use these properties separately or use a shorthand property
border
to combine these into one line (giving the three values in any order). These two things are equivalent:figure { border-style: solid; border-width: 3px; border-color: red; } figure { border: solid red 3px; }
line-height
- Controls the amount of vertical space each line of text takes up (which you might know as “leading”). For example, “
line-height: 1.5;
” sets the spacing between single- and double-spaced (and is probably a good default value. font-family
- Sets the font for the text. You should give a list of fonts that are tried in-order until the browser finds one available on the user's system. There are five generic font families and your list must end with one of them since it's guaranteed to work. For example, “
font-family: "Garamond", "Times", serif;
”.
CSS Box Model
There are several properties in CSS that are often grouped together as box properties which control the way browsers lay out the page using the CSS box model.
These properties might not be immediately intuitive, but aren't too difficult. Suppose we have an element on the page (like a <h2>Element Contents</h2>
). Here are the parts of its “box” when drawn:
So, this CSS code:
h2 { padding: 1em; border: medium dashed black; background-color: grey; }
… will make the <h2>
have a grey background, with 1em
of space between the text and the border. The space inside the border (even if it's invidible because you don't have one) is covered with the background colour and is controlled by the padding
properties.
If we want more space separating this element (and its border) from the stuff around it, we would have to increase the margin
values.
It's easy to mistake margin and padding, especially when there is no border or background colour. If you are trying to change the space around an element (especially when the browser's default CSS has some space there), try setting both margin and padding to zero and work from there.
ul { margin: 0em; padding: 0em; }
CSS Units
You probably noticed the measurement 1em
above. The em
is a unit of (length) measurement in CSS. Here are some common units that need a little explanation:
em
- The current font size: if the current text is 12 point then this will be 12 points. Another unit, an
ex
is half the text size. px
- One screen pixel (dot) on the display. (Note: for some very high resolution devices, real “pixels” are very small, so this length is adjusted to be close to the size of a pixel on a traditional display.)
mm
- A millimetre. There are also units for centimetre, inch, etc. (Note: this is the browsers best guess, but might be innacurate depending on the scaling of the display/projector/phone/etc. For example if you display your screen on a projector, the “millimetre” suddenly becomes much larger.)
As much as possible, I suggest you specify measurements using em
s and ex
s. These are the only units that don't require some kind of note describing when they are a lie. If you think in em
s, you will be thinking properly about the way a page might scale depending on the current screen/font/whatever size.
The one exception is when dealing with bitmapped images: they are inherently sized in pixels, so that probably makes the most sense.
p { line-height: 1.5em; } blockquote { margin-left: 2em; border-left: 0.25em solid black; } img#ourlogo { width: 120px; height: 160px; float: left; margin-left: 1em; }
Example
Here is an example page that we can style with some of the properties above:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8" /> <title>CSS Properties</title> <link rel="stylesheet" href="css-prop.css" /> </head> <body> <h1>CSS Properties</h1> <h2>Goals</h2> <p>This is a page that we're using to demonstrate various CSS properties and techniques. Because of that, it's probably going to be ugly.</p> <h2>Results</h2> <p>Yup, it's turning out rather ugly, but it's important to demonstrate some CSS stuff. Here are some of the new things:</p> <ul> <li>more CSS properties</li> <li>the box model</li> <li>the units of length used</li> </ul> </body> </html>
… and a CSS to go with it:
body { font-family: "Helvetica", sans-serif; } h1 { text-align: center; font-weight: bold; background-color: silver; color: teal; padding: 0.25em; } h2 { border: medium dotted teal; font-weight: normal; padding: 0.1em; }
You can look at that page with the stylesheet yourself, or in my browser it looks like this:
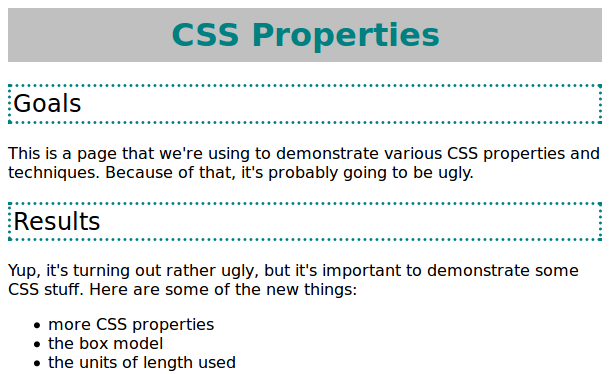
When creating a stylesheet for a page, remember that you're always adding to the browser's default style. In this example, setting font-weight: bold;
for the <h1>
probably doesn't have any effect since the default is bold, but it doesn't hurt either. In this example, we removed the bold font from the <h2>
.
The width of the border was specified as medium
but could also have been a length like 0.1em
.
Try experimenting with CSS on this page (or one you have created). There is no substitute for trying out some stuff and seeing how it affects the page.